Lottie
airbnb 에서 만든 Lottie 사용법입니다.
Android 에서는 많이 써봤는데, 이번에는 Javascript 에서 사용방법을 남겨보려합니다.
간단 사용법 : (feat: lottie-player.js)
단순 html 태그로 사용 가능합니다.
우선 LottieFiles 에서 원하는 Animation 을 찾습니다.
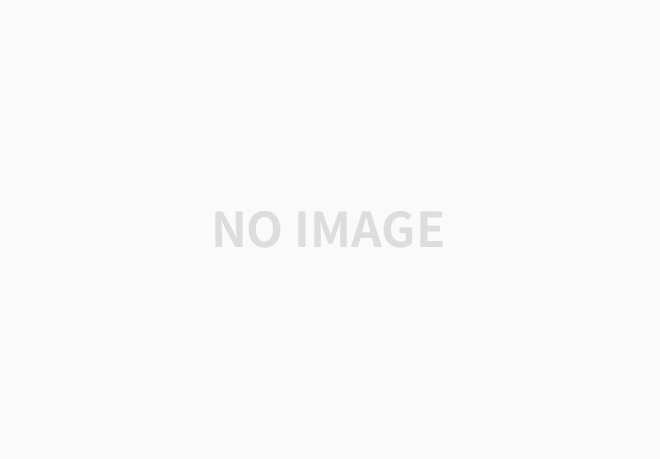
아이템을 클릭하면 아래와 같은 팝업이 뜹니다.
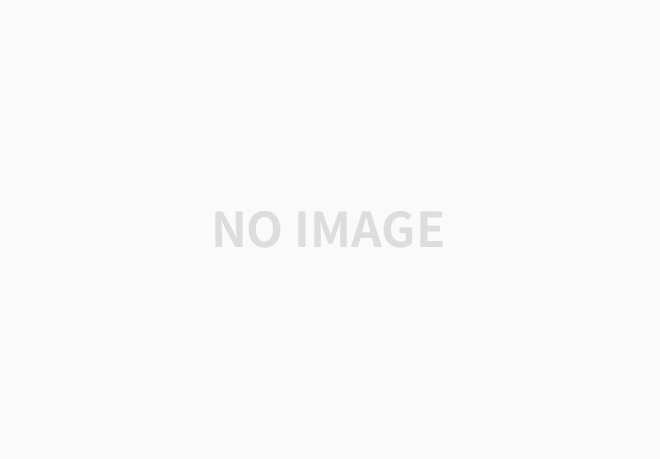
아래로 스크롤해서 <html> 버튼을 찾아 클릭합니다.
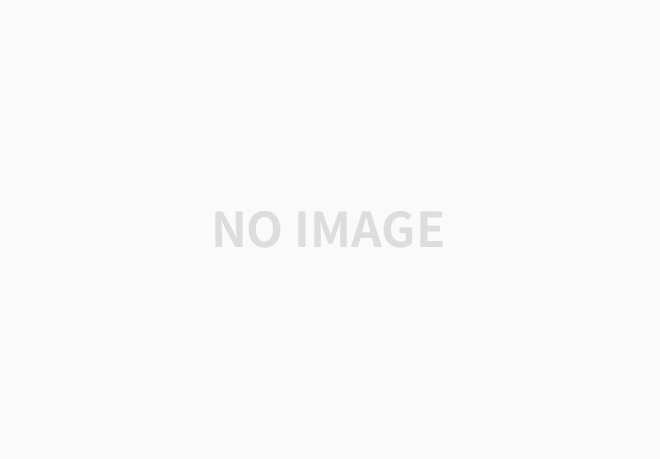
새로운 페이지가 뜹니다. 그 하단에 코드가 있습니다.
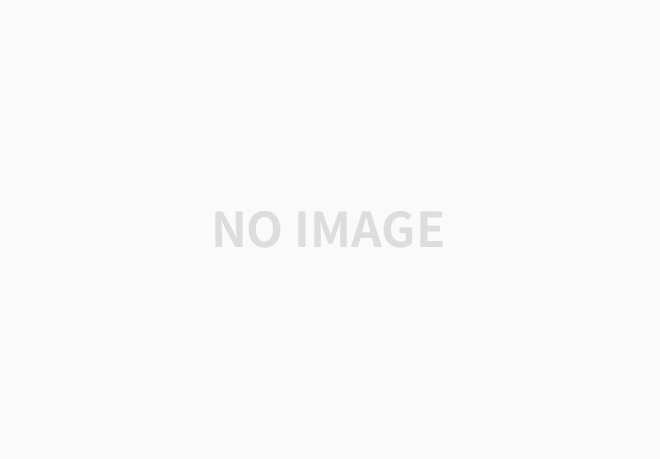
저걸 복사해서 html 코드에 집어 넣으면,
아래와 같이 나옵니다.
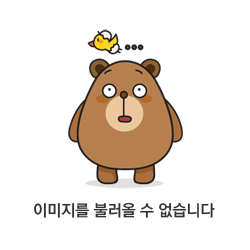
자동 재생 여부, 재생 속도, 크기, 반복여부, 컨트롤 표시 여부 등 tag 에서 설정 가능합니다.
<lottie-player src="https://assets1.lottiefiles.com/packages/lf20_fglzy9ns.json"
background="transparent" speed="1" style="width:300px;height:300px;" loop controls autoplay></lottie-player>
Javascript 에서 컨트롤
버튼을 클릭했거나, ajax callback 이거나, 내가 원하는 순간에 animation이 발생하고 사라지게 하기 위해서는
<script src="https://cdnjs.cloudflare.com/ajax/libs/bodymovin/5.5.9/lottie.min.js"></script>
lottie.js 를 사용합니다.
우선 결과먼저 보여드리면,
마지막 버튼을 클릭하면 lottie animation 이 실행됩니다.
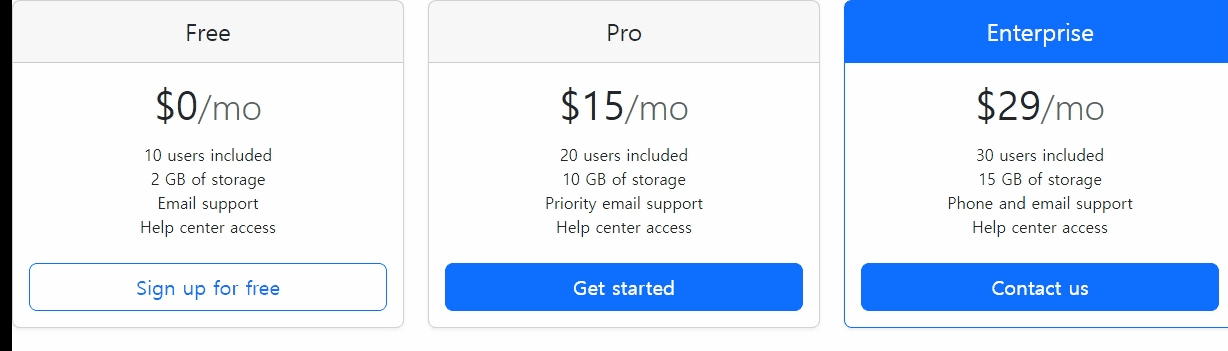
예제 디자인을 포함한 전체 소스 입니다.
<!-- CSS only -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-0evHe/X+R7YkIZDRvuzKMRqM+OrBnVFBL6DOitfPri4tjfHxaWutUpFmBp4vmVor" crossorigin="anonymous">
<script src="https://cdnjs.cloudflare.com/ajax/libs/bodymovin/5.5.9/lottie.min.js"></script>
<div class="row row-cols-1 row-cols-md-3 mb-3 text-center">
<div class="col">
<div class="card mb-4 rounded-3 shadow-sm">
<div class="card-header py-3">
<h4 class="my-0 fw-normal">Free</h4>
</div>
<div class="card-body">
<h1 class="card-title pricing-card-title">$0<small class="text-muted fw-light">/mo</small></h1>
<ul class="list-unstyled mt-3 mb-4">
<li>10 users included</li>
<li>2 GB of storage</li>
<li>Email support</li>
<li>Help center access</li>
</ul>
<button type="button" class="w-100 btn btn-lg btn-outline-primary">Sign up for free</button>
</div>
</div>
</div>
<div class="col">
<div class="card mb-4 rounded-3 shadow-sm">
<div class="card-header py-3">
<h4 class="my-0 fw-normal">Pro</h4>
</div>
<div class="card-body"">
<h1 class="card-title pricing-card-title">$15<small class="text-muted fw-light">/mo</small></h1>
<ul class="list-unstyled mt-3 mb-4">
<li>20 users included</li>
<li>10 GB of storage</li>
<li>Priority email support</li>
<li>Help center access</li>
</ul>
<button type="button" class="w-100 btn btn-lg btn-primary">Get started</button>
</div>
</div>
</div>
<div class="col">
<div class="card mb-4 rounded-3 shadow-sm border-primary">
<div class="card-header py-3 text-white bg-primary border-primary">
<h4 class="my-0 fw-normal">Enterprise</h4>
</div>
<div class="card-body">
<div style="width: 100%;height: 180px;z-index: 999;position: absolute;" id="lottie-test" class="lottie" data-animation-path="animation/" data-anim-loop="true" data-name="ninja"></div>
<h1 class="card-title pricing-card-title">$29<small class="text-muted fw-light">/mo</small></h1>
<ul class="list-unstyled mt-3 mb-4">
<li>30 users included</li>
<li>15 GB of storage</li>
<li>Phone and email support</li>
<li>Help center access</li>
</ul>
<button type="button" class="w-100 btn btn-lg btn-primary" onClick="testStart()">Contact us</button>
</div>
</div>
</div>
</div>
<script>
var lottieAnim = lottie.loadAnimation({
container: document.getElementById("lottie-test"), // the dom element that will contain the animation
renderer: 'svg',
loop: false,
autoplay: false,
path: 'assets/lottie/pop.json' // the path to the animation json
});
function testStart(){
lottieAnim.stop();
lottieAnim.play();
}
</script>
// path: 'assets/lottie/pop.json' // 로컬 path 일 경우.
path: 'https://assets1.lottiefiles.com/packages/lf20_fglzy9ns.json' // URL 도 가능
container 는 lottie animation 을 render 할 (위에서는 'svg') 를 담을 컨테이너를 말합니다.
위에서는 id="lottie-test" 의 div 입니다.
로컬에 json 파일들을 저장해 둬도 되지만, path 에 url 을 직접 입력해줘도 됩니다.
url 은 아까 LottieFiles 사이트의 아래 부분에서 복사하면 됩니다.
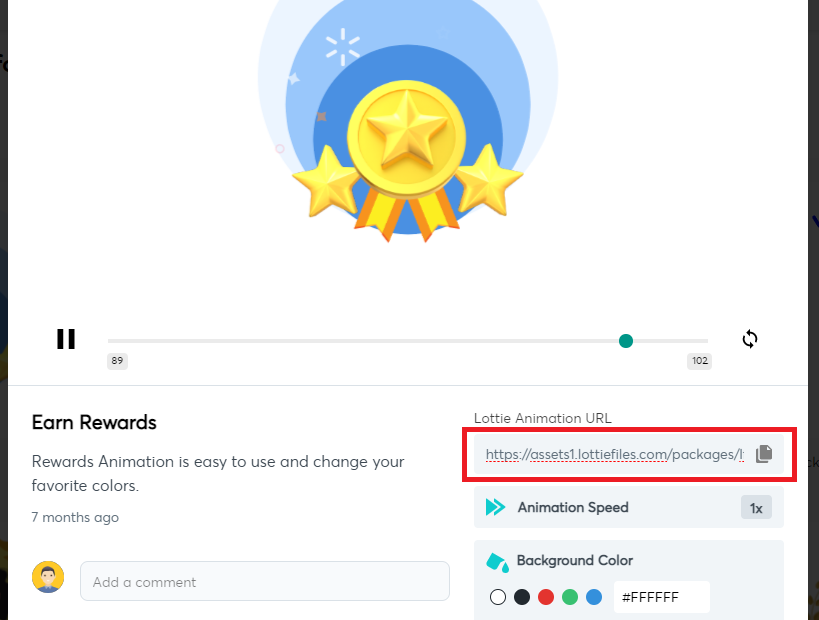
Event 도 추가할 수 있습니다.
예를들어 animation 이 한번 재생되고 사라지도록 하려면, (loop 는 false이고)
lottieAnim.onComplete = function() {
lottieAnim.destroy();
}
그 외 이벤트나 메소드들은 아래 참고하시면 됩니다~
<Lottie Document 참고>
Usage
animation instances have these main methods:
- anim.play()
- anim.stop()
- anim.pause()
- anim.setLocationHref(locationHref) -- one param usually pass as location.href. Its useful when you experience mask issue in safari where your url does not have # symbol.
- anim.setSpeed(speed) -- one param speed (1 is normal speed)
- anim.goToAndStop(value, isFrame) first param is a numeric value. second param is a boolean that defines time or frames for first param
- anim.goToAndPlay(value, isFrame) first param is a numeric value. second param is a boolean that defines time or frames for first param
- anim.setDirection(direction) -- one param direction (1 is normal direction.)
- anim.playSegments(segments, forceFlag) -- first param is a single array or multiple arrays of two values each(fromFrame,toFrame), second param is a boolean for forcing the new segment right away
- anim.setSubframe(flag) -- If false, it will respect the original AE fps. If true, it will update as much as possible. (true by default
- anim.destroy()
lottie has 8 main methods:
- lottie.play() -- with 1 optional parameter name to target a specific animation
- lottie.stop() -- with 1 optional parameter name to target a specific animation
- lottie.setSpeed() -- first param speed (1 is normal speed) -- with 1 optional parameter name to target a specific animation
- lottie.setDirection() -- first param direction (1 is normal direction.) -- with 1 optional parameter name to target a specific animation
- lottie.searchAnimations() -- looks for elements with class "lottie"
- lottie.loadAnimation() -- Explained above. returns an animation instance to control individually.
- lottie.destroy() -- To destroy and release resources. The DOM element will be emptied.
- lottie.registerAnimation() -- you can register an element directly with registerAnimation. It must have the "data-animation-path" attribute pointing at the data.json url
- lottie.setQuality() -- default 'high', set 'high','medium','low', or a number > 1 to improve player performance. In some animations as low as 2 won't show any difference.
Events
- onComplete
- onLoopComplete
- onEnterFrame
- onSegmentStart
you can also use addEventListener with the following events:
- complete
- loopComplete
- enterFrame
- segmentStart
- config_ready (when initial config is done)
- data_ready (when all parts of the animation have been loaded)
- DOMLoaded (when elements have been added to the DOM)
- destroy
'React,Node,JQuery,js' 카테고리의 다른 글
[Javascript] Json Copy. DeepCopy (2) | 2023.01.28 |
---|---|
[Javascript] JSON Object key, value 가져오기 (0) | 2023.01.28 |
[Lodash] 유용한 자바스크립트 라이브러리 : Javascript Library (0) | 2022.06.27 |
virtual dom 이란? (0) | 2021.06.23 |
[Nodejs] session 사용하기 (0) | 2021.01.15 |
댓글